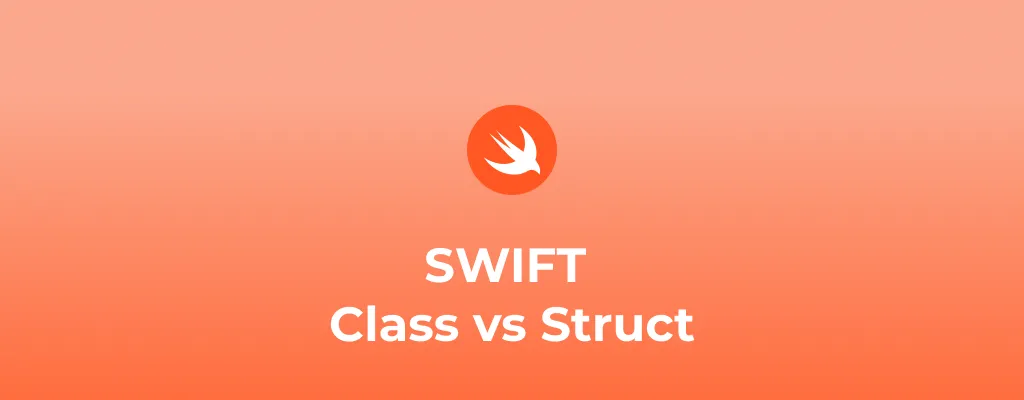
What’s the Difference Between Struct and Class in Swift?
If you're diving into Swift development, you've probably encountered the terms "struct" and "class" when working with data and objects. These two fundamental constructs have some key differences that can impact how your code behaves. Let's break it down in simple terms.
Definition
-
Struct: Think of a struct as a lightweight container. It's perfect for holding simple data types like integers, floats, and booleans. Structs are value types, meaning they are copied when passed around.
Example:
// Define a struct for representing a point in 2D space
struct Point {
var x: Double
var y: Double
}
// Create instances of Point
var pointA = Point(x: 1.0, y: 2.0)
var pointB = pointA // Creates a copy of pointA
pointA.x = 3.0
print(pointA) // Output: Point(x: 3.0, y: 2.0)
print(pointB) // Output: Point(x: 1.0, y: 2.0) - pointB remains unchanged
-
Class: A class, on the other hand, is more heavyweight. It's used for creating objects that have reference semantics. This means when you pass a class instance around, you're passing a reference to the same object.
Example:
// Define a class for representing a person
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
// Create instances of Person
var personA = Person(name: "Alice", age: 30)
var personB = personA // Both personA and personB reference the same object
personA.age = 31
print(personA.age) // Output: 31
print(personB.age) // Output: 31 - personB reflects the change made to personA
Mutability
Struct: Instances of structs are immutable by default. If you want to modify a property of a struct, you need to create a new instance with the changes.
Class: Classes are mutable by default. You can change their properties directly since you're working with references to the same object.
Inheritance
Struct: Structs don't support inheritance. You can't create a new struct based on an existing one.
Class: Classes support inheritance, allowing you to create subclasses that inherit properties and methods from a parent class.
Copy vs. Reference
Struct: When you pass a struct to a function or assign it to another variable, a copy is made. Changes in one instance won't affect others.
Class: With classes, you're working with references. Multiple variables can point to the same object, so changes made through one reference affect all others pointing to the same object.
Performance
Struct: Due to their copy-on-assignment behavior, structs can be more memory-efficient for small data types.
Class: Classes might consume more memory due to references, especially when dealing with a large number of objects.
Use Cases
Struct: Use structs for simple data modeling, like representing coordinates, dates, or other value-based entities.
Class: Classes are ideal for more complex objects, such as view controllers, network managers, and model objects that need to be shared and mutated.
In conclusion, the choice between struct and class in Swift depends on your specific needs. Structs are great for simplicity and avoiding shared state, while classes provide more flexibility when you need reference semantics and inheritance. Understanding these differences is key to writing efficient and maintainable Swift code. Happy coding!