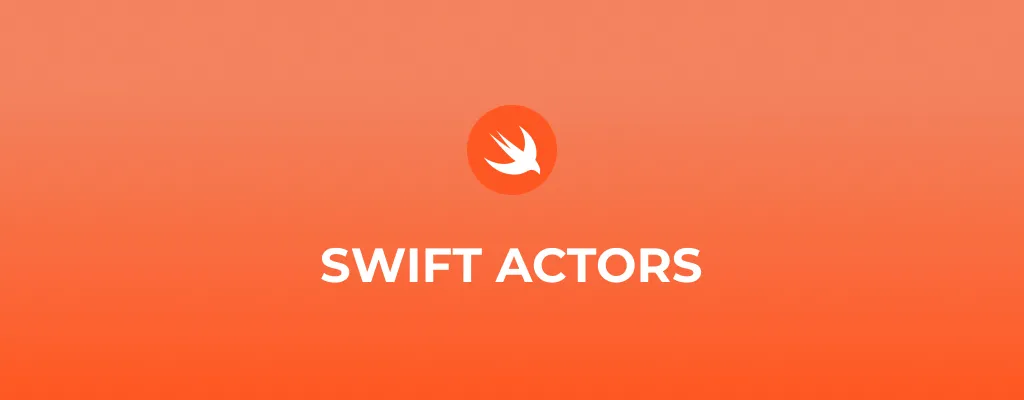
Simplifying Concurrent Programming with Swift Actors
Introduction
Concurrent programming is essential for developing high-performance and responsive applications. However, it can be challenging to write concurrent code that is safe, efficient, and free from data races. In Swift, the introduction of actors in version 5.5 addresses these challenges by providing a simplified and safer approach to managing mutable states in concurrent environments. In this blog post, we will explore a use case that demonstrates the limitations of traditional concurrent programming without locks, discuss how locks can mitigate the issues, and finally showcase how actors offer an elegant solution.
Swift Actors
Actors are an important feature introduced in Swift that provides a powerful mechanism for managing concurrent code and ensuring thread safety. Here are a few key things you need to know about actors:
- Concurrency and Isolation: Actors enable safe and efficient concurrent programming by ensuring that only one task can access its mutable state at a time. This isolation eliminates data races and makes it easier to reason about the behavior of concurrent code.
- Encapsulation and Messaging: Actors encapsulate their state and behavior, and interactions with an actor are done through message passing. This means that other code can only access an actor's state by sending messages to it, and the actor processes these messages in a serial and ordered fashion.
- Protection and Synchronization: Actors handle the synchronization internally, so you don't need to manually manage locks or other synchronization primitives. The actor system automatically serializes access to an actor's mutable state, ensuring thread safety without the risk of deadlocks or race conditions.
- Async/Await: Actors work seamlessly with Swift's
async
/await
concurrency model. You can define actor methods asasync
and useawait
to wait for the completion of asynchronous tasks within the actor. This simplifies writing asynchronous code and makes it more readable. - Efficiency and Performance: Actors employ various optimization techniques to minimize overhead and improve performance. They leverage structured concurrency to efficiently manage task execution and eliminate unnecessary context switches, making concurrent code faster and more efficient.
- Actor Isolation: Actors are inherently isolated entities, meaning that their internal state is protected from direct external access. This isolation enables actors to provide a clear boundary between different parts of a system, promoting modular and maintainable code.
Use Case: Bank Account Operations
Consider a scenario where multiple threads or tasks need to perform deposit and withdrawal operations on a shared bank account concurrently. Let's explore how traditional concurrent programming approaches, without using locks, can lead to data races and inconsistencies.
Thread unsafe code
In this approach, we create a BankAccount
class without locks, which can result in data races. The code might look like this:
import Foundation
class BankAccount {
var balance: Double = 0.0
func deposit(amount: Double) {
balance += amount
}
func withdraw(amount: Double) {
if amount <= balance {
balance -= amount
} else {
print("Insufficient funds!")
}
}
}
let account = BankAccount()
let group = DispatchGroup()
DispatchQueue.concurrentPerform(iterations: 100) { _ in
group.enter()
DispatchQueue.global().async {
account.deposit(amount: 10.0)
group.leave()
}
group.enter()
DispatchQueue.global().async {
account.withdraw(amount: 5.0)
group.leave()
}
}
group.wait()
print("Final balance: \(account.balance)")
// Final balance: 500.0
// Final balance: 470.0
In this code, multiple threads or tasks can simultaneously access and modify the balance
property without coordination. This lack of synchronization can lead to data races, where multiple threads concurrently modify shared state, resulting in inconsistent and incorrect values. The final balance may not reflect the correct sum of all the deposits and withdrawals made.
Concurrent Code with Locks
To address the issues of data races and inconsistencies, we can introduce locks to synchronize access to the mutable state. Let's modify the BankAccount
example to incorporate locks:
import Foundation
class BankAccount {
var balance: Double = 0.0
private let lock = NSLock()
func deposit(amount: Double) {
lock.lock()
balance += amount
lock.unlock()
}
func withdraw(amount: Double) {
lock.lock()
if amount <= balance {
balance -= amount
} else {
print("Insufficient funds!")
}
lock.unlock()
}
}
let account = BankAccount()
let group = DispatchGroup()
DispatchQueue.concurrentPerform(iterations: 100) { _ in
group.enter()
DispatchQueue.global().async {
account.deposit(amount: 10.0)
group.leave()
}
group.enter()
DispatchQueue.global().async {
account.withdraw(amount: 5.0)
group.leave()
}
}
group.wait()
print("Final balance: \(account.balance)")
// Final balance: 500.0
// Final balance: 500.0
In this modified code, we use an NSLock
instance called lock
to ensure exclusive access to the mutable state. By acquiring the lock before modifying the balance
property and releasing it afterward, we prevent multiple threads from accessing it simultaneously. This synchronization guarantees consistent and correct results.
Concurrent Code with Actors
Swift 5.5 introduced actors to address the challenges associated with traditional concurrent programming. Actors simplify the management of mutable state by encapsulating it within the actor's scope and ensuring exclusive access. Let's see how the BankAccount
example is transformed using actors:
actor BankAccount {
var balance: Double = 0.0
func deposit(amount: Double) {
balance += amount
}
func withdraw(amount: Double) {
if amount <= balance {
balance -= amount
} else {
print("Insufficient funds!")
}
}
}
let account = BankAccount()
Task {
await withTaskGroup(of: Void.self) { taskGroup in
for _ in 0..<100 {
taskGroup.addTask {
await account.deposit(amount: 10.0)
}
taskGroup.addTask {
await account.withdraw(amount: 5.0)
}
}
await taskGroup.waitForAll()
}
let finalBalance = await account.balance
print("Final balance: \(finalBalance)")
}
// Final balance: 500.0
// Final balance: 500.0
With actors, there's no need for manual locks or synchronization. The BankAccount
actor ensures that only one operation can access its mutable state at any given time, eliminating the risk of data races and simplifying concurrent programming.
In the modified code, the BankAccount
class is defined as an actor using the actor
keyword. The deposit and withdraw methods can be called concurrently on different threads or tasks, and the actor automatically handles the serialization and synchronization of access to its mutable state.
Conclusion
Swift actors provide a modern and simplified approach to concurrent programming by eliminating the complexities associated with manual synchronization using locks. By encapsulating mutable state within actors, Swift ensures safe and efficient concurrent access without the risk of data races.
In our use case, the BankAccount
example illustrated the limitations of traditional concurrent programming approaches without actors, leading to the introduction of locks. However, with the introduction of actors, the code became more concise, readable, and less prone to concurrency-related issues. Swift actors empower developers to focus on writing correct and performant concurrent code while reducing the potential for errors.
With Swift 5.5 and the advent of actors, concurrent programming in Swift has taken a significant step forward. It opens up new possibilities for building scalable, responsive, and thread-safe applications with ease. By embracing actors, developers can simplify their code, enhance productivity, and unlock the full potential of concurrent programming in Swift.
Xcode playground https://github.com/LeTadas/SwiftActorsPlayground